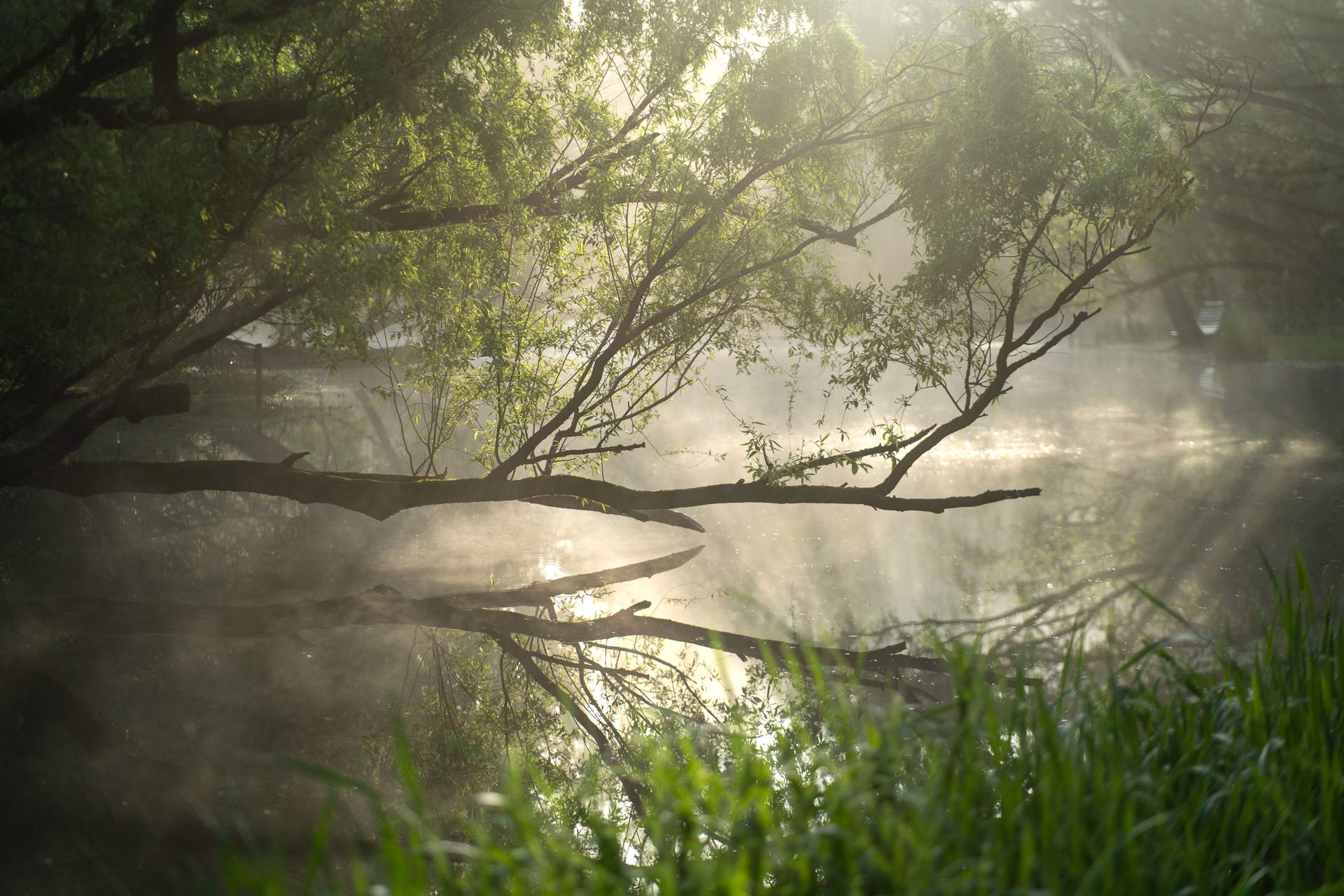
5 Ways to Preload Images in Web Applications
With the increasingly rich API of web applications and improved device performance, user experience (UX) can be optimized by preloading images. This article introduces several ways to preload images in web applications.
Principles
Web applications function based on HTTP/HTTPS. Preloading involves writing the resources needed for a web page into the browser or disk cache, allowing the page to read from the cache directly when the resources are needed.
When Do We Need Preloading
Preloading is particularly useful in the following scenarios:
- Opening a popup window with a background image via user interaction
- Switching between pages, which may be time-consuming due to the need to load new images or cause a flicker if not preloaded
- Knowing in advance that a particular static image will be needed in the near future
- …
Implementing Preloading
Method 1:<link preload>
“Preload” is a keyword for the rel attribute of the <link>
element, indicating that the browser should pre-fetch and cache the resource, as there’s a high chance the user will need it during the current browsing session.
Example:
1 | <head> |
Insert a <link>
element with rel="preload"
, as="image"
, and an href
attribute for the resource URL within the header. Multiple types of static resources can be preloaded with the <link preload>
element (refer to the as attribute documentation for more information).
Implement Preload Through JavaScript
1 | const preload = (src: string) => { |
For Next.js users, the built-in next/head
component allows easy modification of the HTML document’s header. Official documentation
1 | import Head from 'next/head' |
You can attach state to your link or preload multiple image resources using an array, depending on how you organize your code logic.
Read more about the specifications and compatibility of the preload attribute inLink types: preload.
Method 2:new Image()
A simpler way to preload images is to create an <img />
element with the new Image()
constructor.
1 | const preloadImage = (src: string) => { |
This code runs eagerly, immediately triggering the browser to retrieve the image resource when preloadImage
is called.
Method 3: XHR
Similar to method 2, we can cache resources using XHR.
1 | const preloadImage = (src: string) => { |
In this example, we didn’t perform any processing on the image resource retrieved via XHR, but you can use URL.createObjectURL()
to employ it.
Method 4: CSS
Using CSS to load resources requires the CSS to be used. This method is less flexible than others listed above.
When the browser parses CSS rules with static resources that are being used, it will load the resources automatically.
1 | .example { |
Method 5: Non-Visible Elements
Inserting an element into HTML will trigger the browser to parse its style and load any required static resources.
1 | const preloadImage = (src: string) => { |
That concludes our introduction to several ways to preload images in web applications. We hope it helps improve your web app UX.
5 Ways to Preload Images in Web Applications