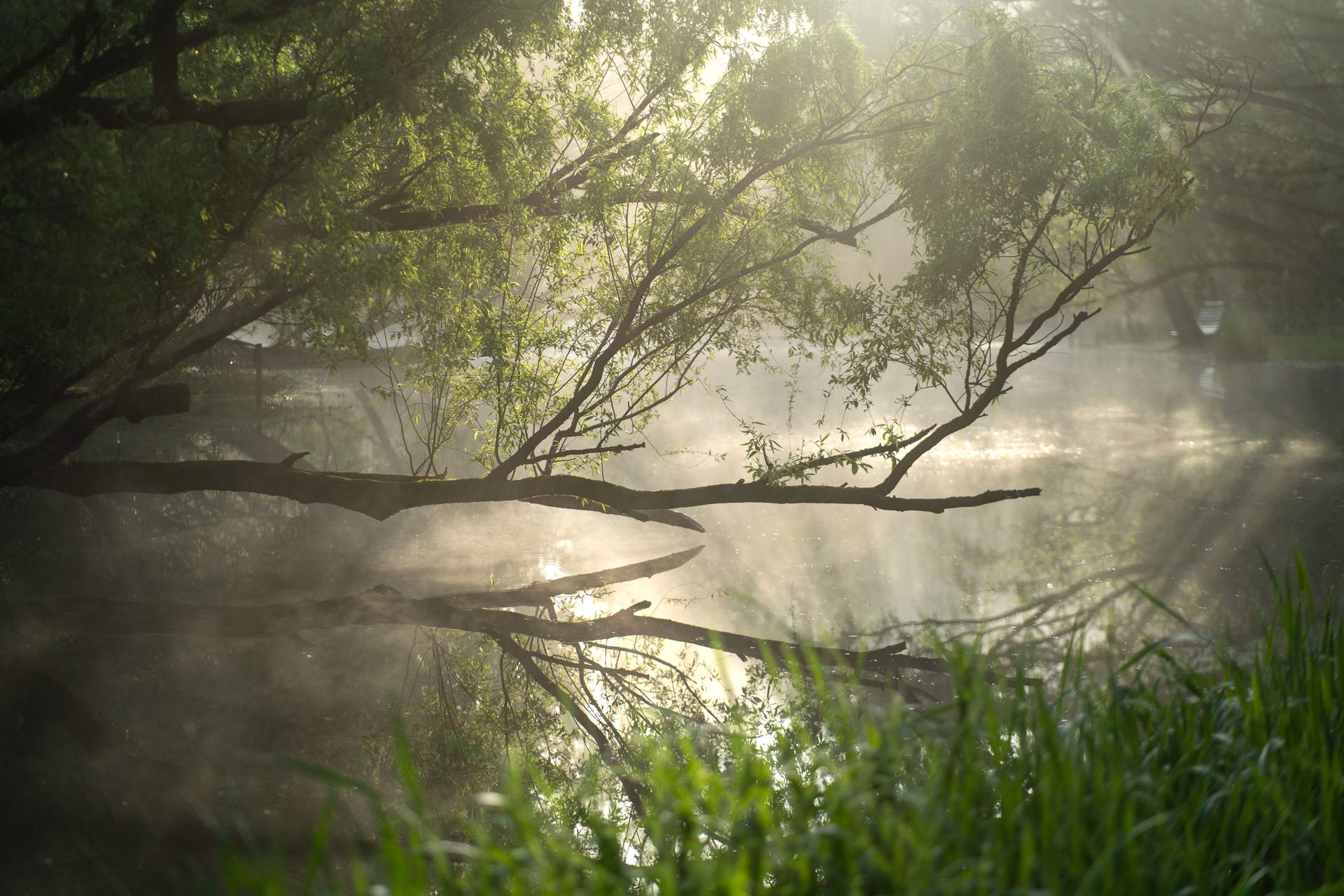
Create a Color Picker With JavaScript
The RGB color mode is a color model formed by combining three primary colors: red, green, and blue. It can be used to describe the colors we see in a digital way, where the intensity of each color is represented by a number between 0 and 255. Therefore, we can know the value of each color in RGB.
Obtaining and processing color data
Step1. Raw Data
color-list
We can obtain some color data and their English names from RGB to Color Name Mapping (Triplet and Hex). Next, let’s process it. Run the following lines of code in the console:
1 | copy( |
Then we can get an array of color data called colorList
, which has been copied to the system clipboard by the program above. The result of this array is as follows:
1 | [ |
Step2. Deduplication
Next, we use the uniqBy
method from lodash to remove duplicates from our color array.copy(_.uniqBy(colorList, 'rgb'))
Step3. Formatting
Separate the RGB values in the color data into individual properties for easy searching and calculation in subsequent steps.
1 | copy( |
Core function: Obtain the color name based on an RGB(A) value.
color-mapping
The basic principle of using the RGB coordinate system to describe colors is already very clear. This method allows us to describe any color in three-dimensional space, where the XYZ coordinates of a color are its RGB values. Through this approach, we can intuitively understand the position of each color in space and their relative positions with respect to one another.
Once we have all the coordinates for each color, we can use basic mathematical algorithms to calculate the distance between any two colors in space. Based on our definition of distance, we can obtain a distance from a target color to every known color and then sort them by size in order to find the closest match.
The core of this algorithm lies in calculating the distance between two colors. We can use a simple formula: The distance between two points equals the square root of the sum of squares of their differences along each dimension. That is, for two points (x1,y1)
and (x2,y2)
on a 2D plane, their distance d
can be expressed as:
d = √[(x1-x2)^2 + (y1-y2)^2]
Similarly, we can express distances between points on a 3D plane as:
d = √[(x1-x2)^2 + (y1-y2)^2 + (z1-z2)^2]
Here, (x1,y1,z1)
and (x2,y2,z22)
represent coordinates for two colors within an RGB coordinate system.
By computing distances between all known colors and our target color, we can identify which one is closest. If it’s necessary to consider non-linear properties within our search for similar colors based on proximity alone - such as when using more advanced algorithms like CIEDE2000 - then these methods may improve accuracy further still; however most cases should be satisfied with simple proximity-based calculations alone.
In this example, the colors obtained from the image also include an alpha channel, which represents transparency. We can obtain its value between 0 and 1 (corresponding to CSS rgba’s alpha value) by dividing alpha
by 255. If it is equal to zero, then regardless of its RGB values, it will be a fully transparent color.
1 | function getColor(r, g, b, a) { |
User Interface
On the user interaction interface, a canvas is placed in the middle area to display the image that needs to be color picked. It is worth noting that our image needs to be fully displayed on the canvas without being stretched or compressed. Using the following method, we can display an image on the canvas.
1 | function init(url) { |
Then we add event listener on the input above our canvas.
1 | onInputFile(e) { |
To simplify user operation costs, we can also monitor page drag and drop events.
1 | onDropFile(e) { |
Note that: In order to achieve file drag and drop, we need to use e.preventDefault()
to prevent the default behavior of the browser for 4 drag and drop events: dragenter
, dragover
, dragleave
, and drop
. (When an image file is dragged into the browser window, the browser will open this image in a new tab by default).
Get Color With Pointer Click
When the pointer clicks on the canvas, we can calculate the position of our click on the canvas through its click event property, and thus obtain the color data of this position.
1 | onCanvasClick(e) { |
Additional feature: Obtain the main color tone of an image.
Color Thief is a JavaScript library used to extract the dominant color palette from an image. It works by sampling pixels of the image, cutting down on the sampled colors, and finally sorting them based on their frequency to determine the main color palette of the image.
Credits
Create a Color Picker With JavaScript